tips
Django Tips #16 Simple Database Access Optimizations
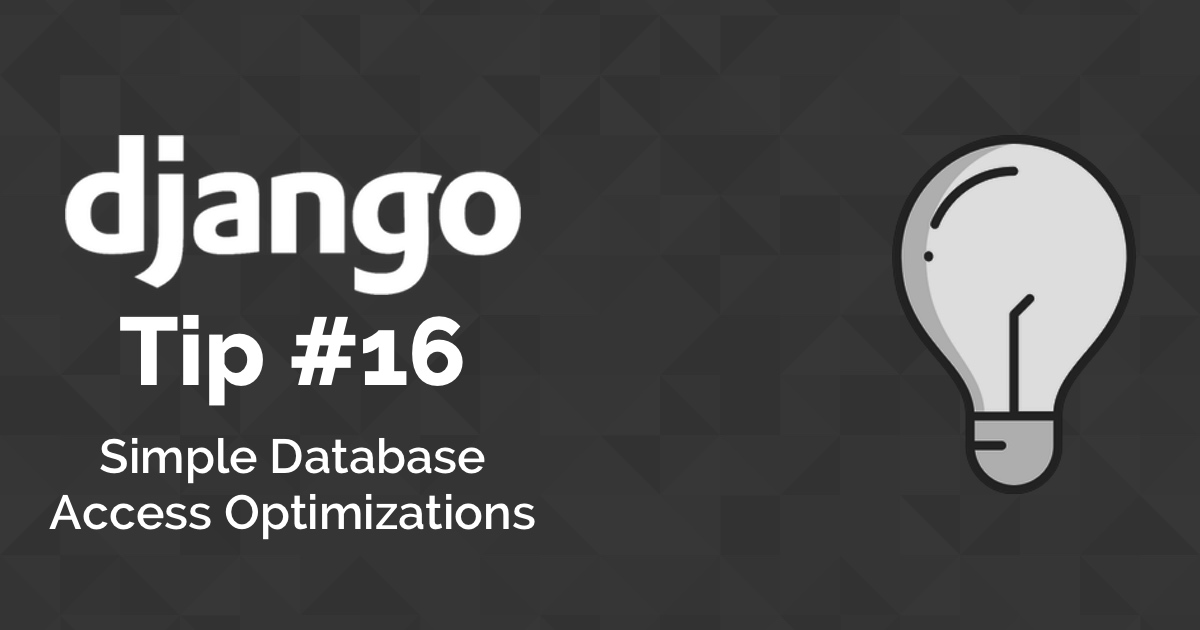
So, I just wanted to share a few straightforward tips about database access optimization. For the most those tips are not something you should go to your code base and start refactoring. Actually for existing code it’s a better idea to profile first (with Django Debug Toolbar for example). But those tips are very easy to start applying, so keep them in mind when writing new code!
tutorial
How to Use Django's Flatpages App
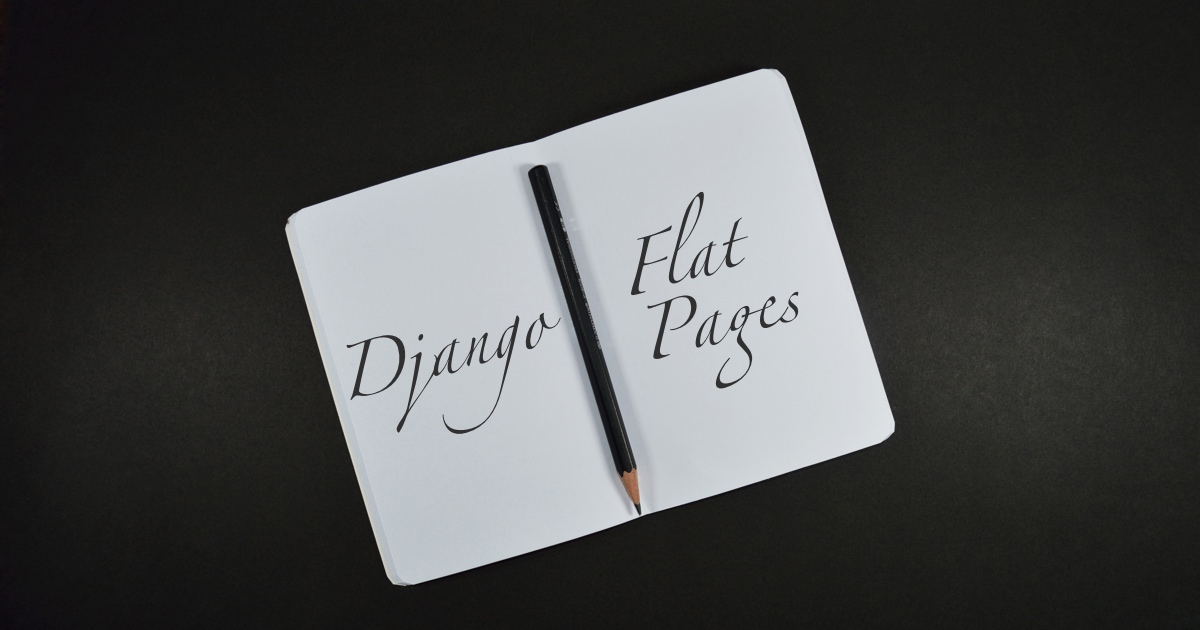
Django ships with a Flatpages application that enables the user to create flat HTML pages and store it in the database. It can be very handy for pages like About, Privacy Policy, Cookies Policy and so on.
article
Estimating the Effort of Development of a Django Application
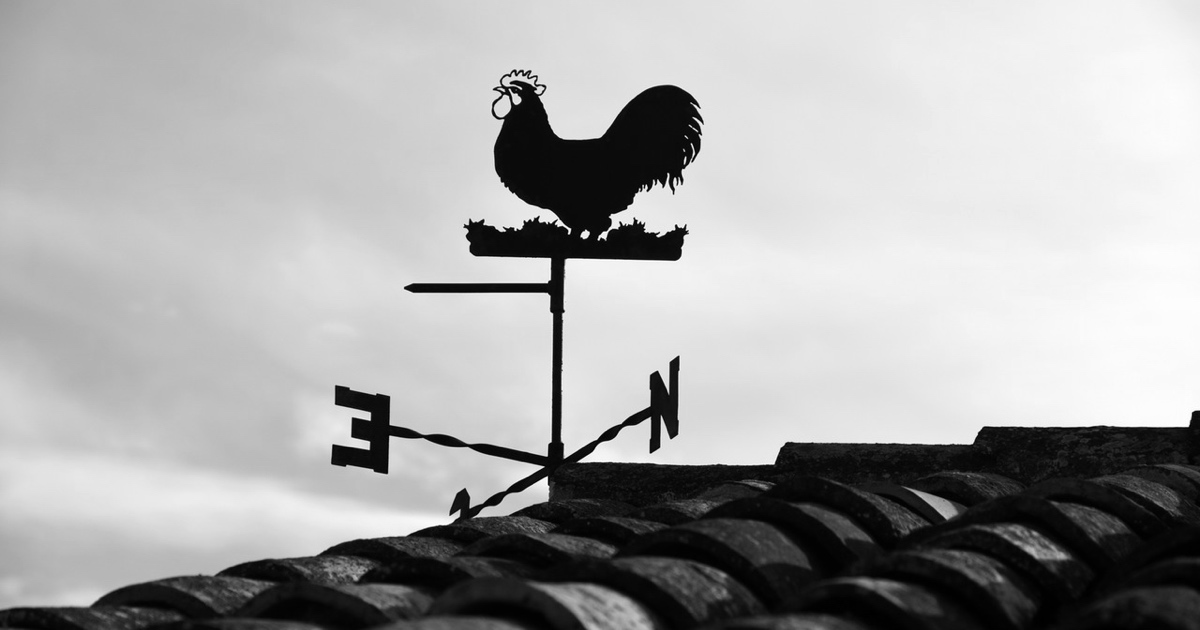
This is a very common problem that every developer will eventually face at some point. Your boss or a client comes with a set of requirements and asks you to estimate how long it will take to implement them.
tips
Django Tips #15 Using Mixins With Class-Based Views
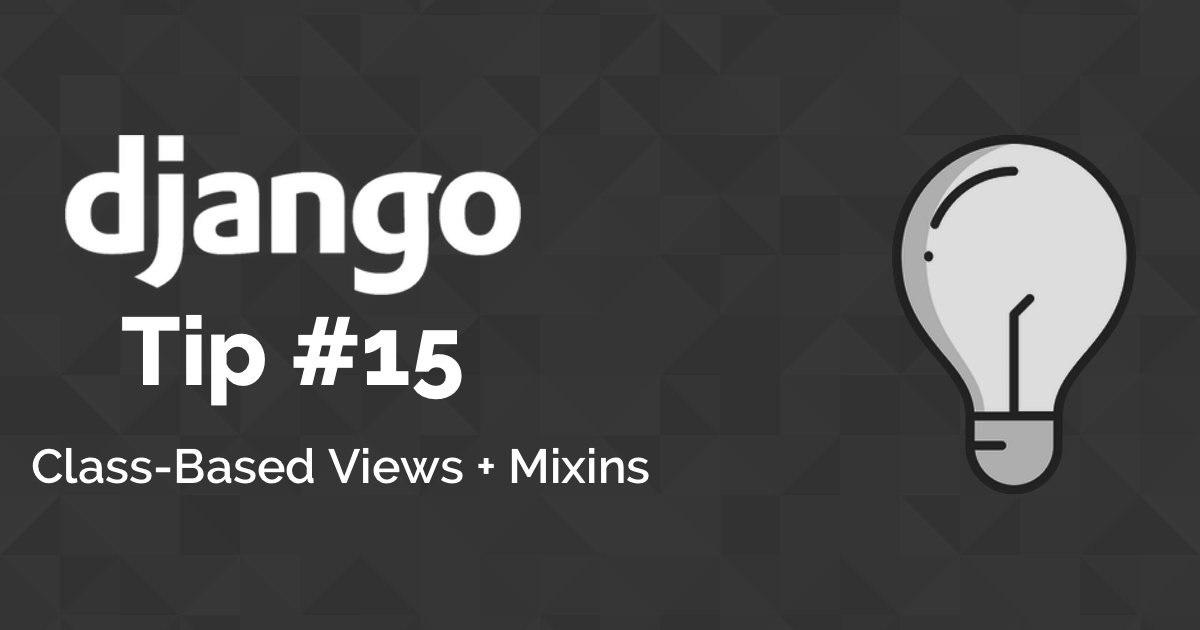
I was reading today the book Two Scoops of Django (affiliate link) by Audrey and Daniel Roy Greenfeld and found some interesting tips about using class-based views with mixins.
tutorial
How to Create a Password Reset View
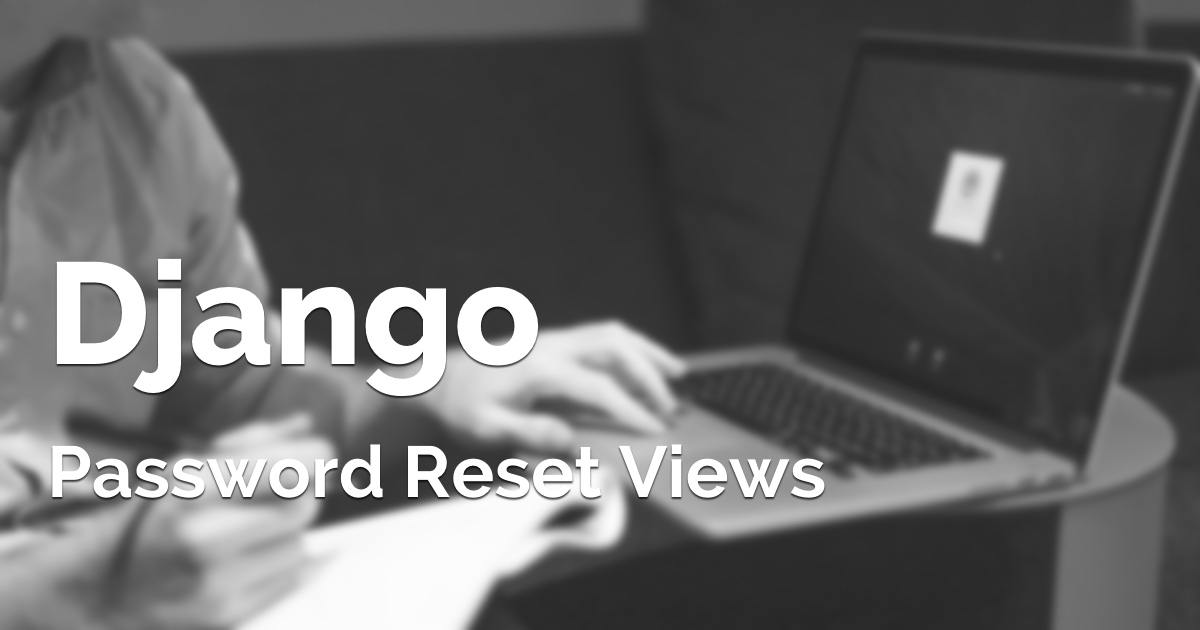
For this short tutorial we will be using the django.contrib.auth
views to add a password reset functionality to your
Django application. The process of reseting passwords involves sending emails. For that matter we will be using console
email backend to debug and check if everything is working. In the end of this tutorial I will also provide resources
to properly configure a prodution-quality email server.
article
Django Shortcuts
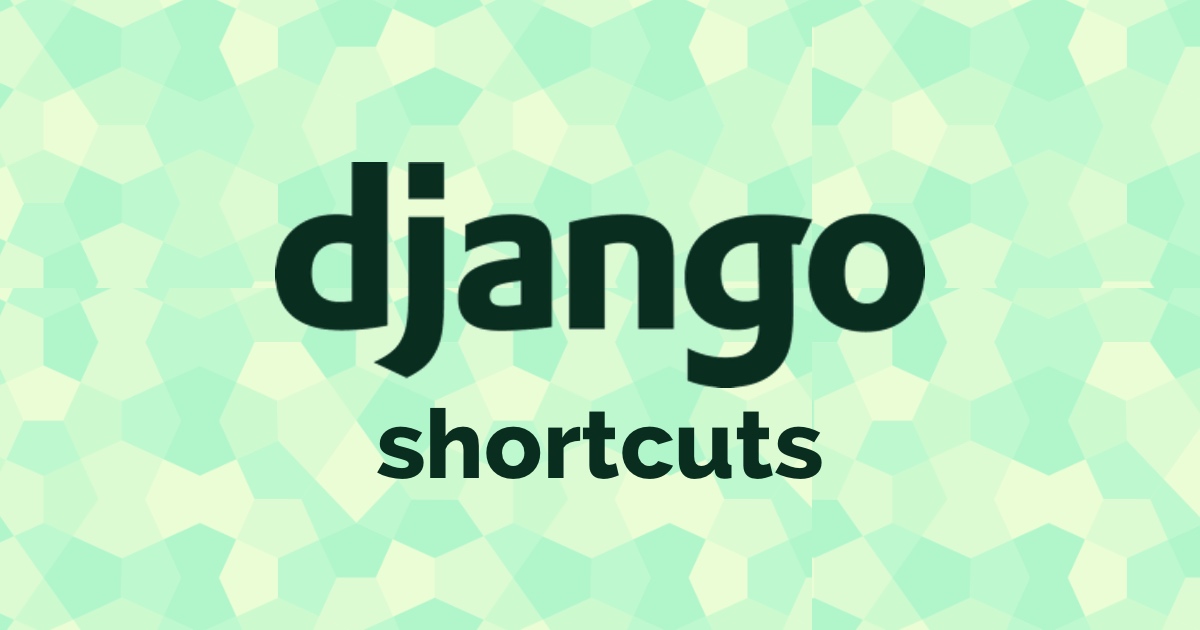
This module is a collection of helper classes generally used in view functions/classes. All the shortcuts are available
in the module django.shortcuts
.
tips
Django Tips #14 Using the Messages Framework
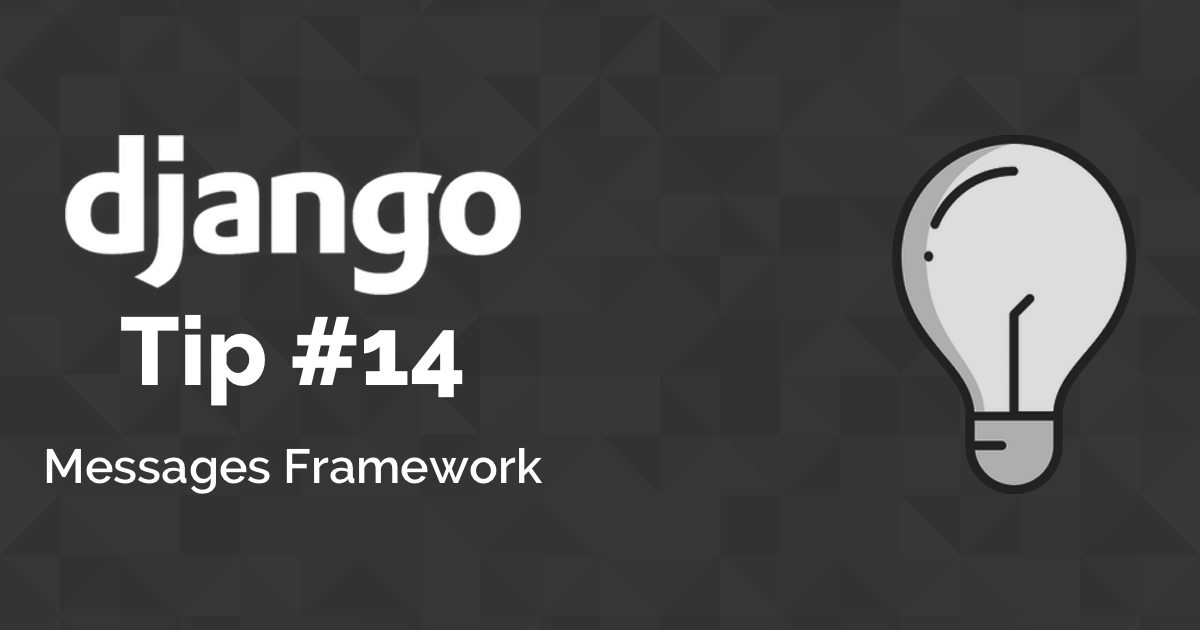
Keeping the users of your application aware of what is going on makes a huge difference in the user experience. If there is something users hate more than slow applications, it is applications that does not communicate with them.
tutorial
How to Work With AJAX Request With Django
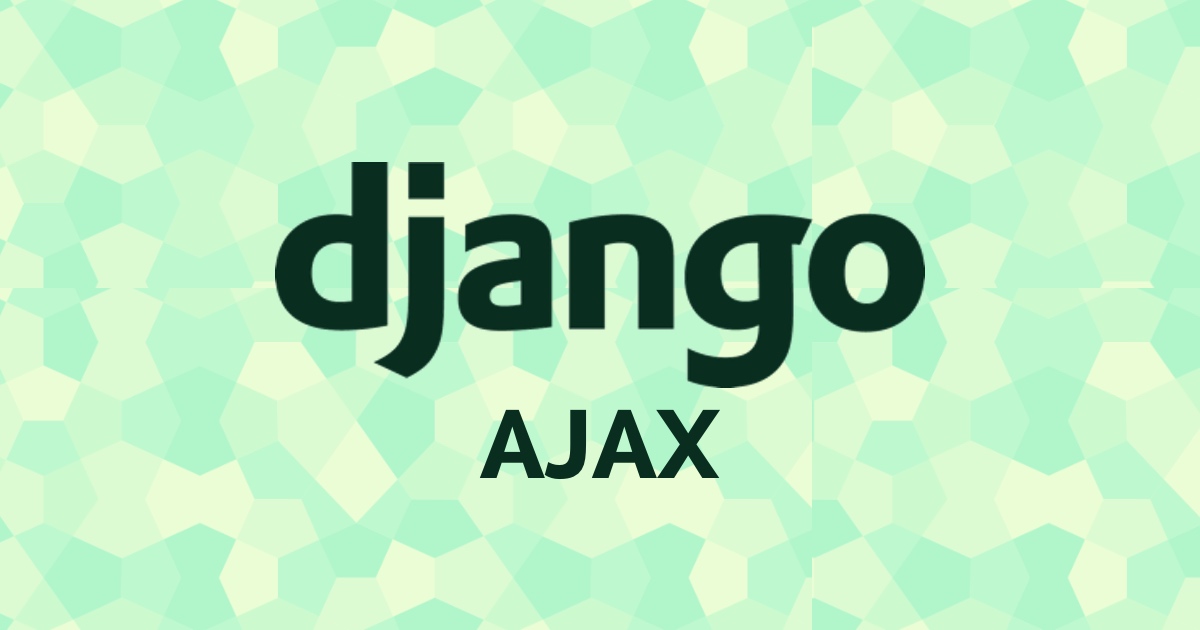
There are many scenarios where you may want to use AJAX requests in your web application. It is a great resource that enables web applications to be faster and more dynamic. For this short tutorial I will be using jQuery to ease the implementation.
tutorial
How to Create a One Time Link
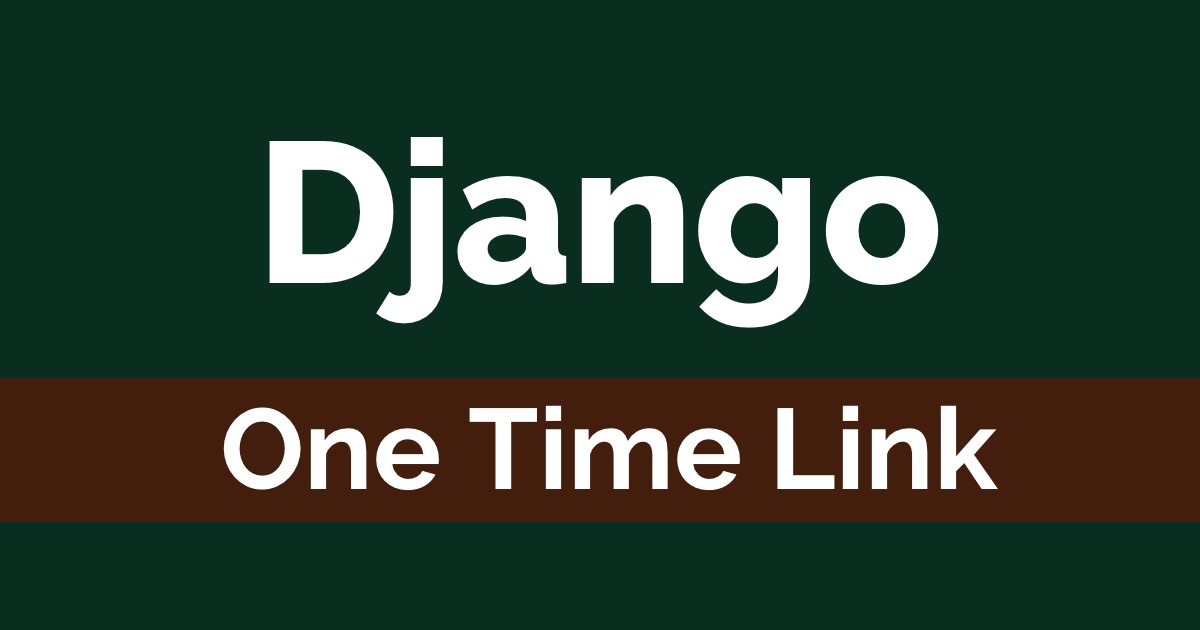
Django uses a very interesting approach to generate the Password reset tokens. I’m not really a security expert, neither I’m very familiar with cryptography algorithms, but it is very safe and reliable.
tips
Django Tips #13 Using F() Expressions
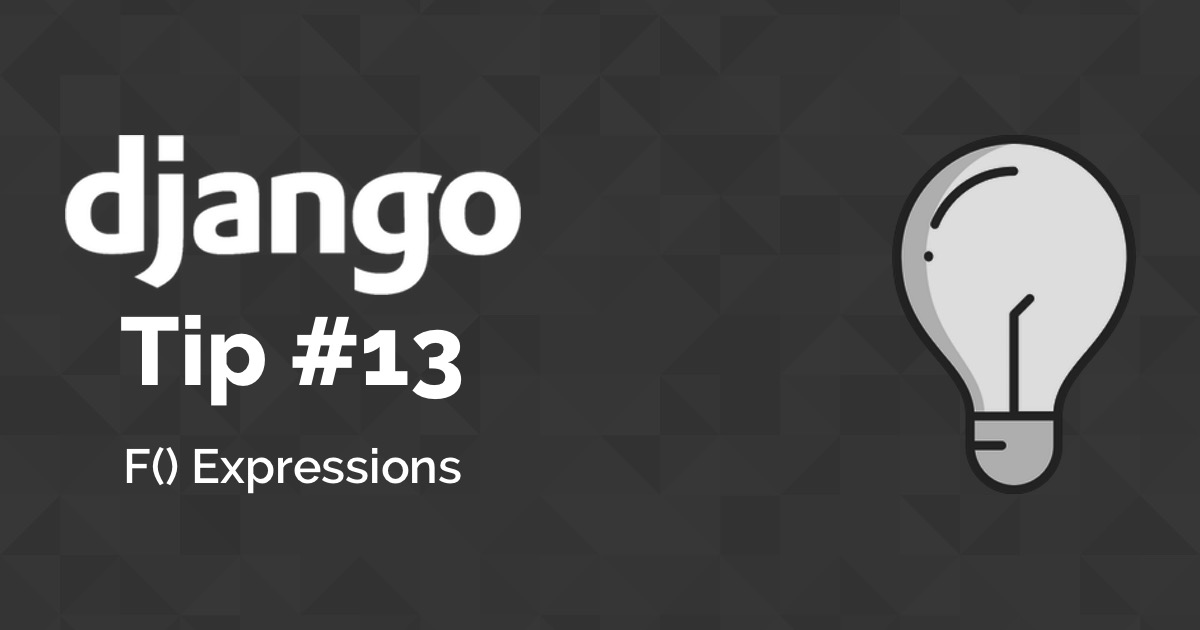
In the Django QuerySet API, F() expressions are used to refer to model field values directly in the database.
Let’s say you have a Product
class with a price
field, and you want to increase the price of all products in 20%.
- ← Previous
- First page
- Page 7 of 11
- Last page
- Next →