tutorial
How to Crop Images in a Django Application
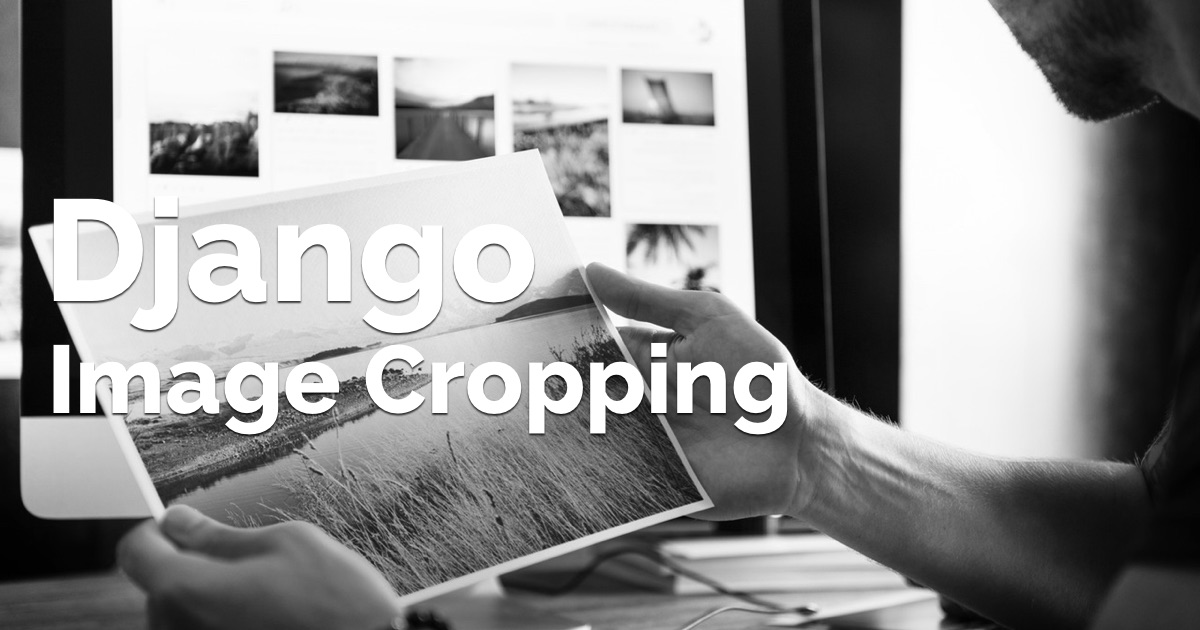
Cropping images is a fairly common use case in a Web application. For example, some applications let you upload a profile picture. In addition, it usually also let you crop and resize the image for a better result. Unfortunately, when dealing with image processing we need to install a few dependencies, both in the front-end and in the back-end.
tutorial
How to Add reCAPTCHA to a Django Site
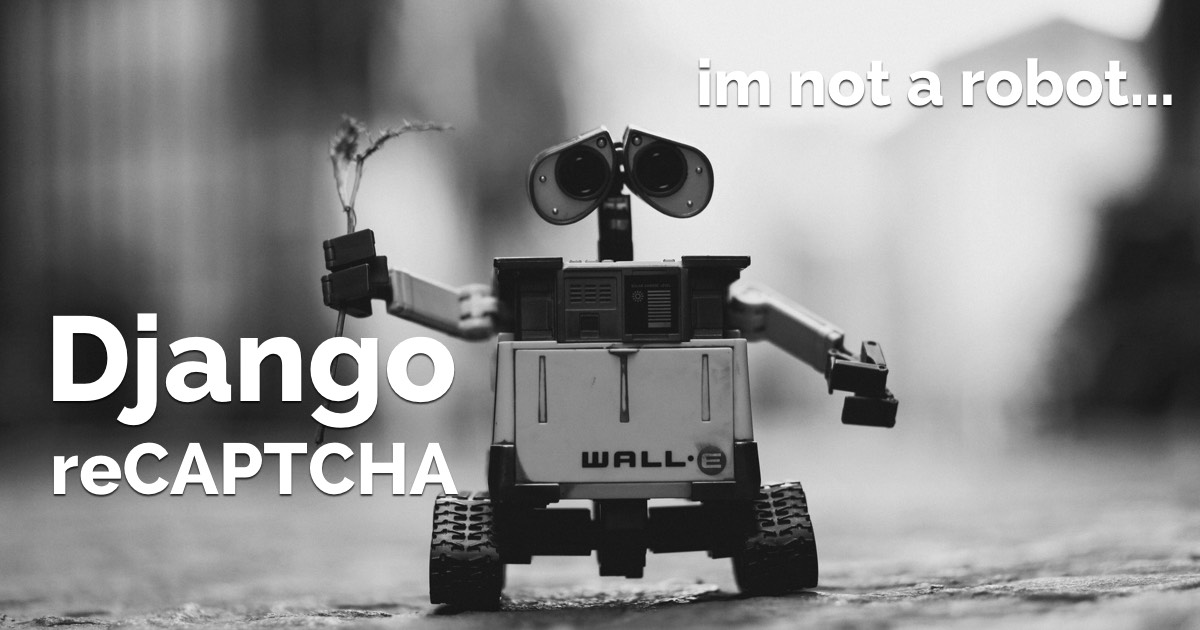
Google’s reCAPTCHA is a very popular solution to protect your application or website against bots and spam. It is
fairly simple to implement. In this tutorial you will find a working example using only built-in libraries, an
alternative using requests
and also an implementation using decorators, to reuse the reCAPTCHA verification across
your application.
tutorial
How to Create User Sign Up View
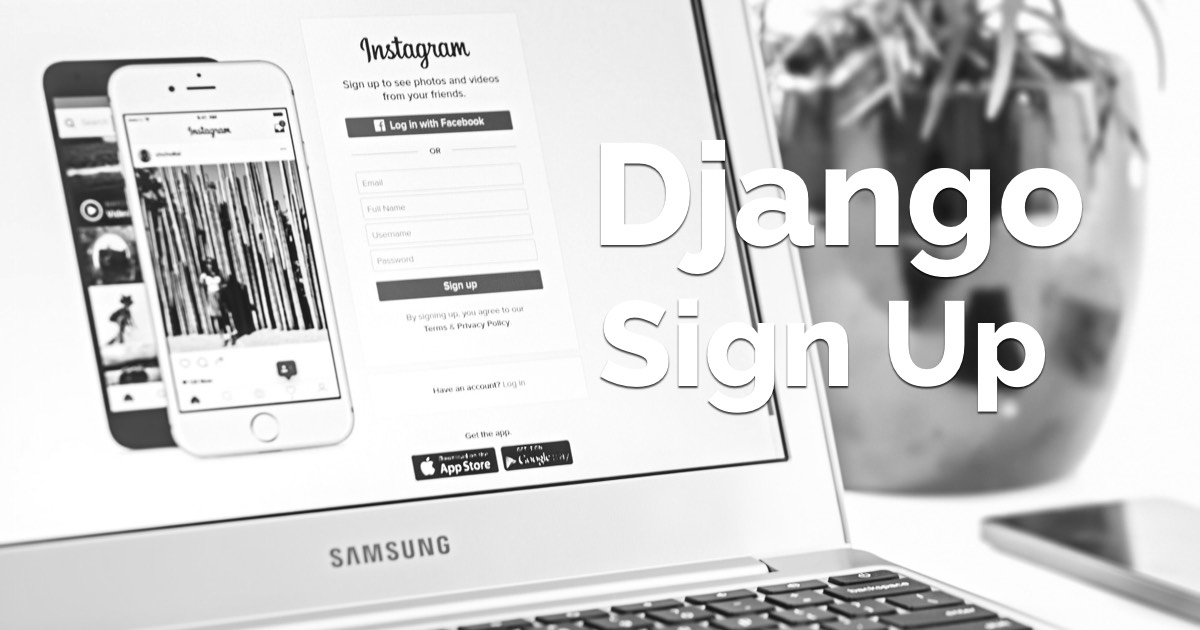
In this tutorial I will cover a few strategies to create Django user sign up/registration. Usually I implement it from scratch. You will see it’s very straightforward.
tutorial
How to Implement Case-Insensitive Username
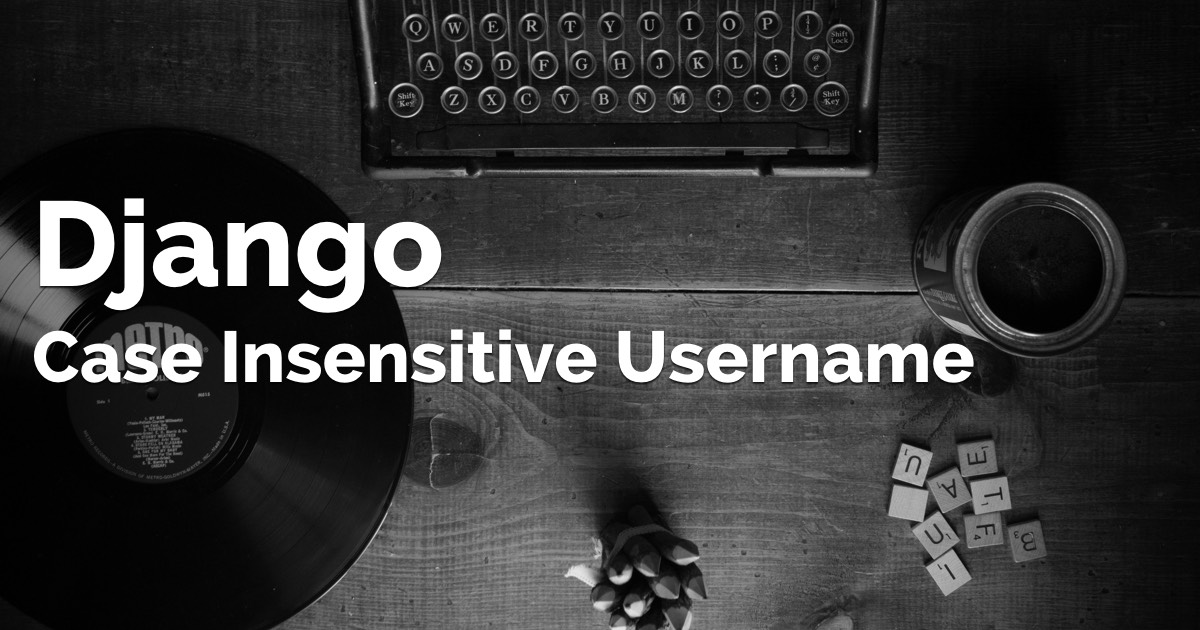
Inspired by a discussion in the How to Extend Django User Model comments, I decided to compile a few options on how to implement a case insensitive authentication using the built in Django User. Thanks to Paul Spiteri for bringing up the question and also to provide a possible solution!
tutorial
How to Create Group By Queries With Django ORM
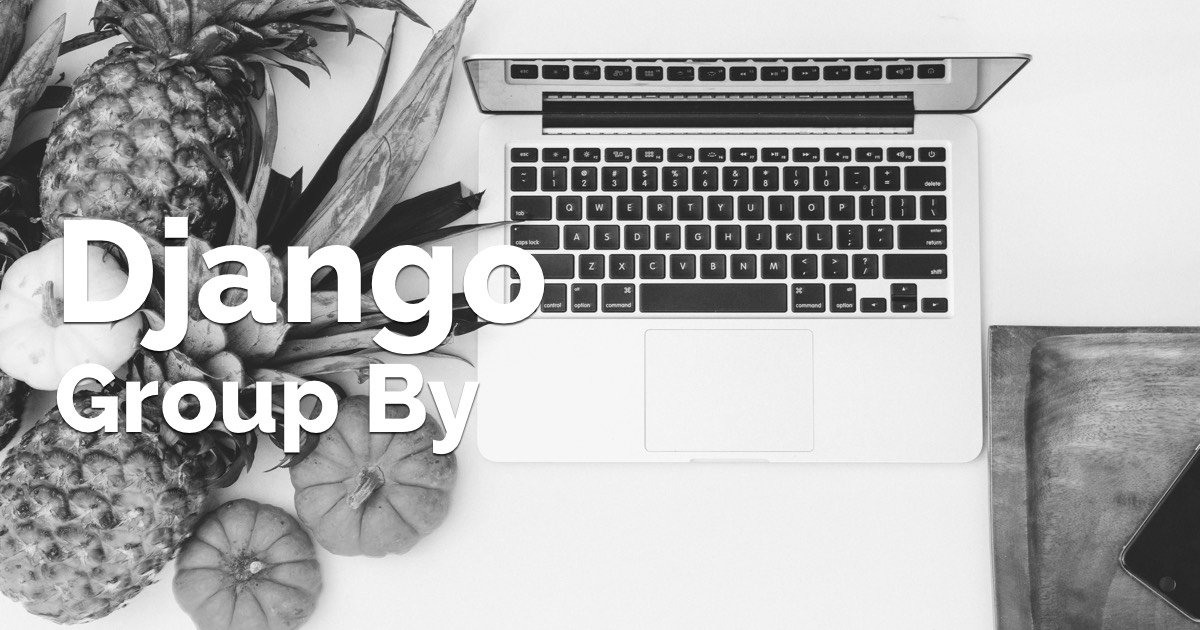
This tutorial is about how to implement SQL-like group by queries using the Django ORM. It’s a fairly common operation, specially for those who are familiar with SQL. The Django ORM is actually an abstraction layer, that let us play with the database as it was object-oriented but in the end it’s just a relational database and all the operations are translated into SQL statements.
tutorial
How to Filter QuerySets Dynamically
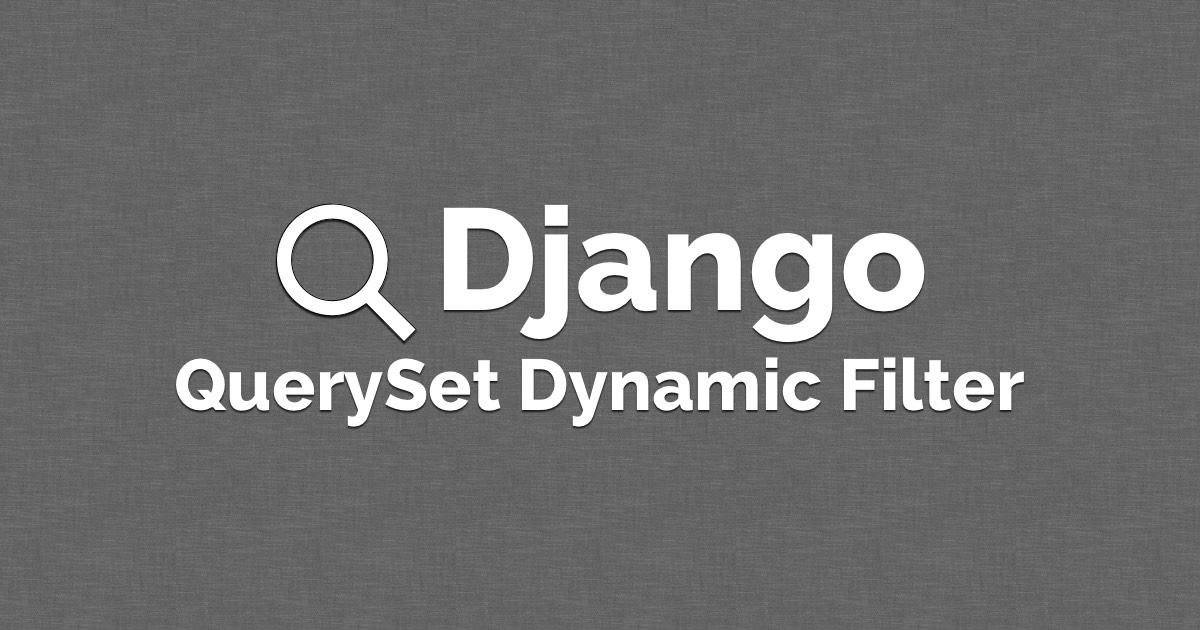
Filtering QuerySets dynamically is a fairly common use case. Sure thing there is a pluggable app to make your life easier. This tutorial is about how to use the django-filter app to add a hassle-free filtering to your views. To illustrate this tutorial I will implement a view to search for users. As usual the code used in this tutorial is available on GitHub. You can find the link in the end of this post.
tutorial
How to Add User Profile To Django Admin
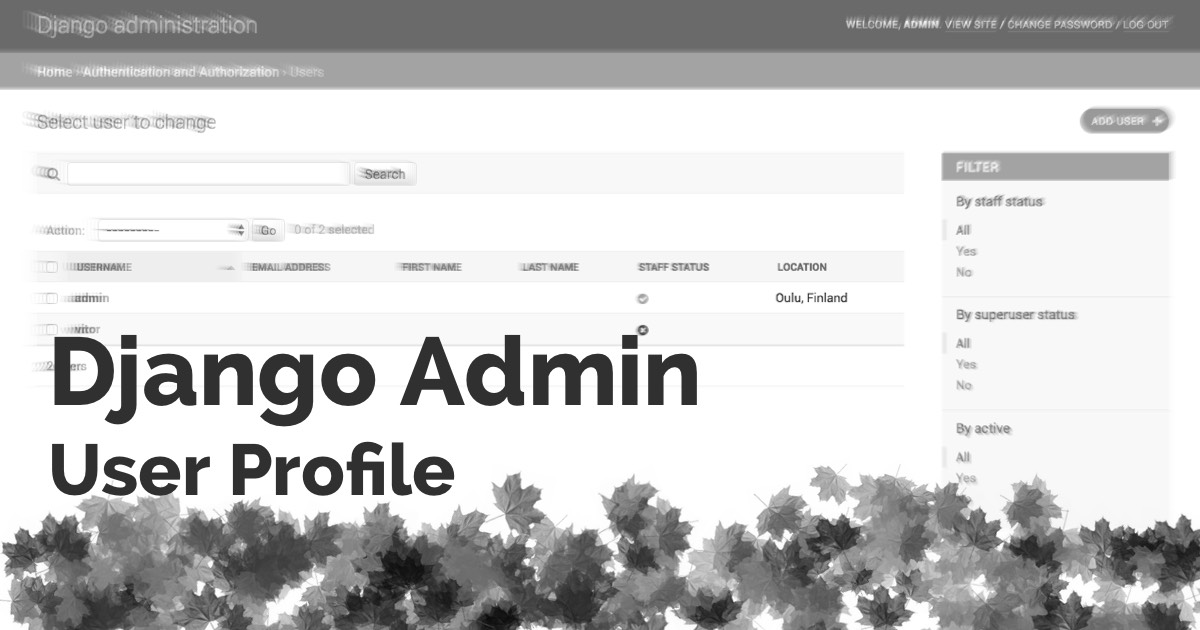
There are several ways to extend the the default Django User model. Perhaps one of the most common way (and also less intrusive) is to extend the User model using a one-to-one link. This strategy is also known as User Profile. One of the challenges of this particular strategy, if you are using Django Admin, is how to display the profile data in the User edit page. And that’s what this tutorial is about.
tutorial
Django Multiple Files Upload Using Ajax
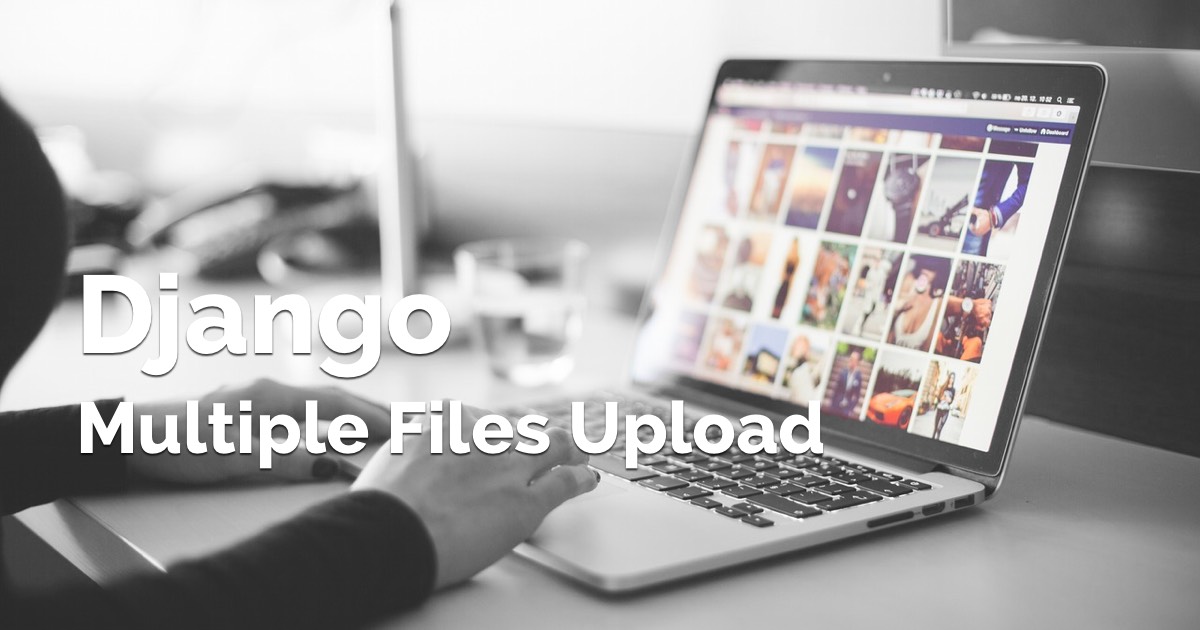
In this tutorial I will guide you through the steps to implement an AJAX multiple file upload with Django using jQuery. For this tutorial we will be using a specific plug-in called jQuery File Upload, which takes care of the server communication using AJAX and also the compatibility with different browsers.
tutorial
How to Implement CRUD Using Ajax and Json
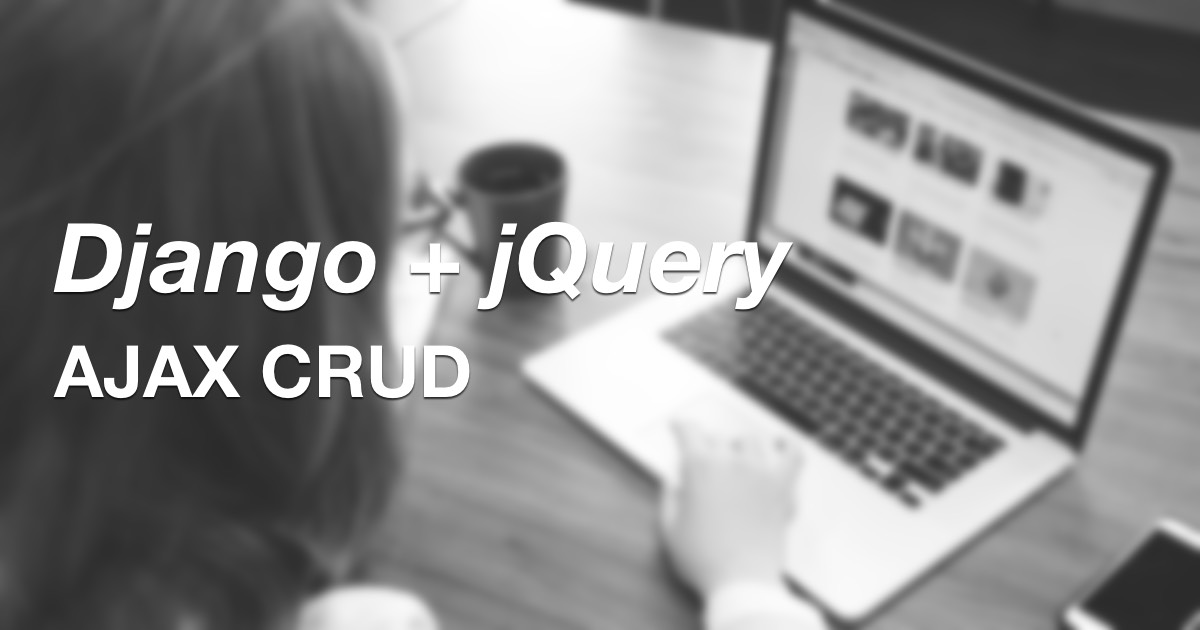
Using Ajax to create asynchronous request to manipulate Django models is a very common use case. It can be used to provide an in line edit in a table, or create a new model instance without going back and forth in the website. It also bring some challenges, such as keeping the state of the objects consistent.
article
Django Under the Hood 2016
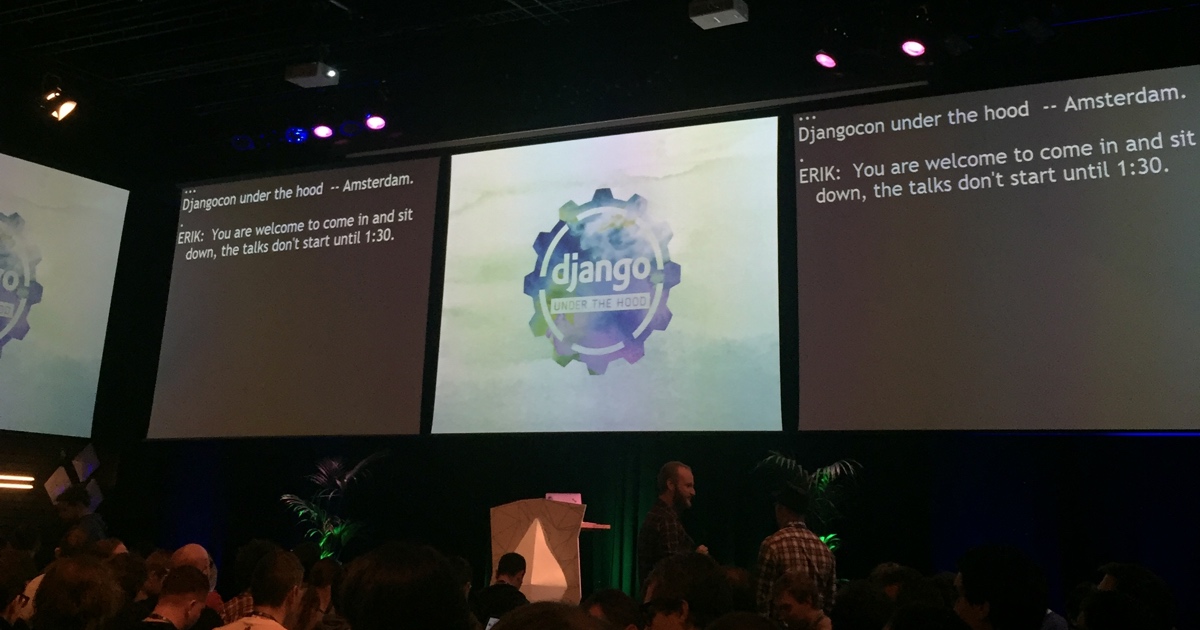
This was the third edition of the Django: Under The Hood (DUTH) conference. Two days of awesome talks and two days of sprints. The conference was organised by members of the Django community, including several members of the Django core team, and in association with the Dutch Django Association.
- ← Previous
- First page
- Page 5 of 11
- Last page
- Next →