tutorial
How to Create Custom Django Management Commands
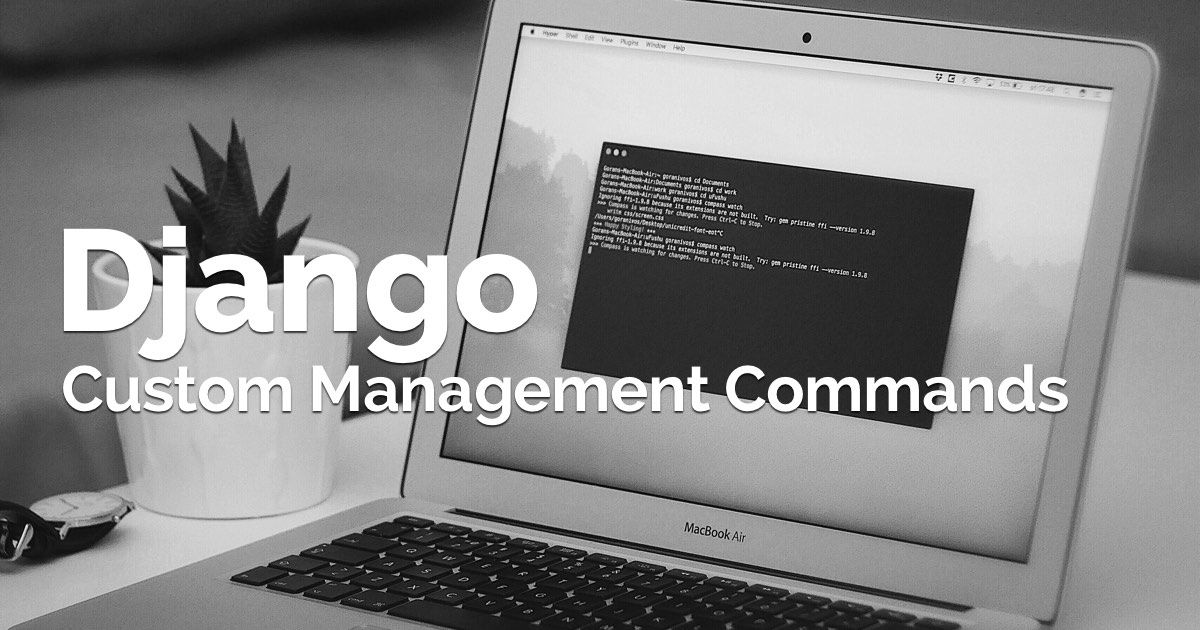
Django comes with a variety of command line utilities that can be either invoked using django-admin.py
or the
convenient manage.py
script. A nice thing about it is that you can also add your own commands. Those management
commands can be very handy when you need to interact with your application via command line using a terminal and it can
also serve as an interface to execute cron jobs. In this tutorial you are going to learn how to code your own commands.
tutorial
How to Use Bootstrap 4 Forms With Django
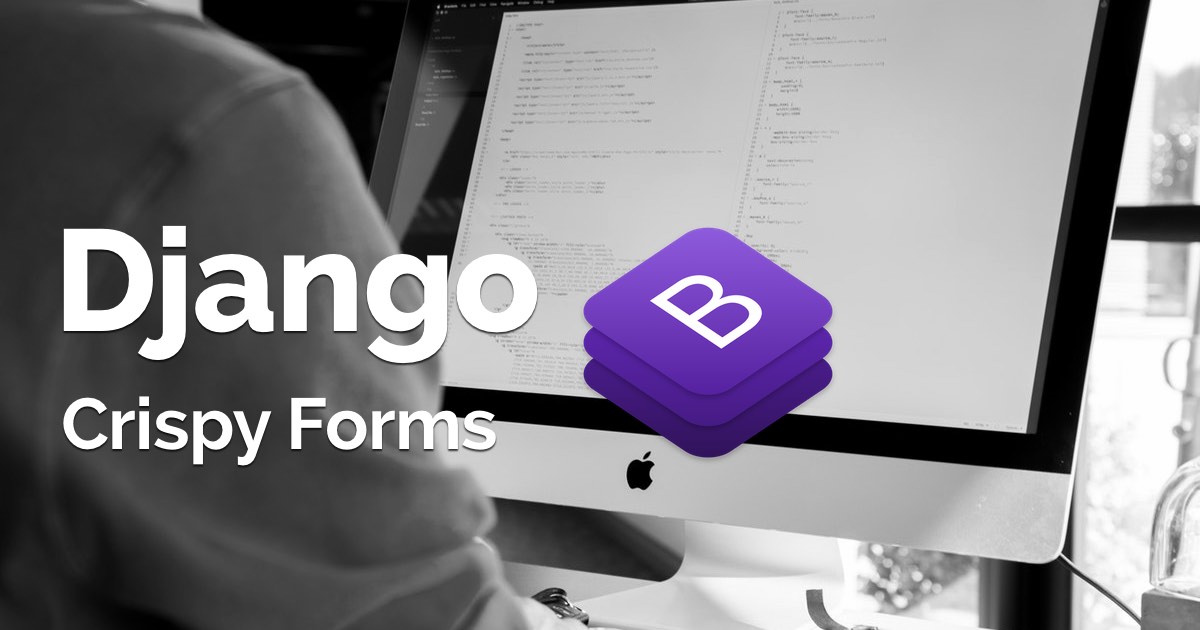
This is a quick tutorial to get you start with django-crispy-forms and never look back. Crispy-forms is a great application that gives you control over how you render Django forms, without breaking the default behavior. This tutorial is going to be tailored towards Bootstrap 4, but it can also be used with older Bootstrap versions as well as with the Foundation framework.
tutorial
How to Integrate Highcharts.js with Django
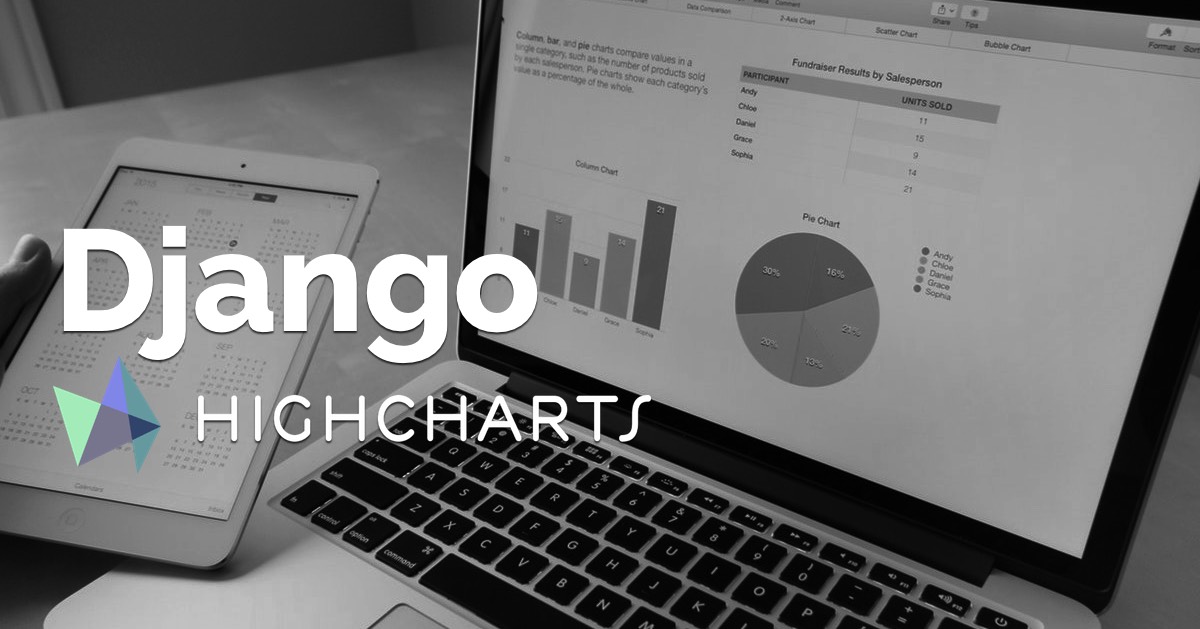
Highcharts is, in my opinion, one of the best JavaScript libraries to work with data visualization and charts out there. Even though Highcharts is open source, it’s a commercial library. It’s free for use in non-commercial applications though.
tips
Django Tips #22 Designing Better Models
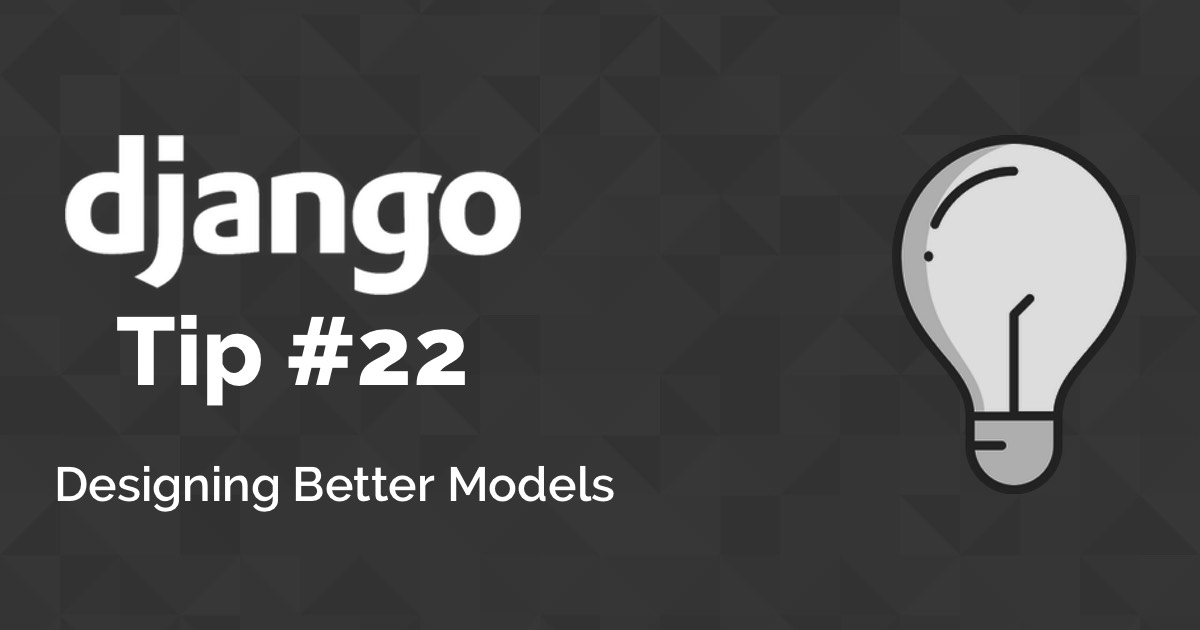
In this post, I will share some tips to help you improve the design of your Django Models. Many of those tips are related to naming conventions, which can improve a lot the readability of your code.
tutorial
How to Use RESTful APIs with Django
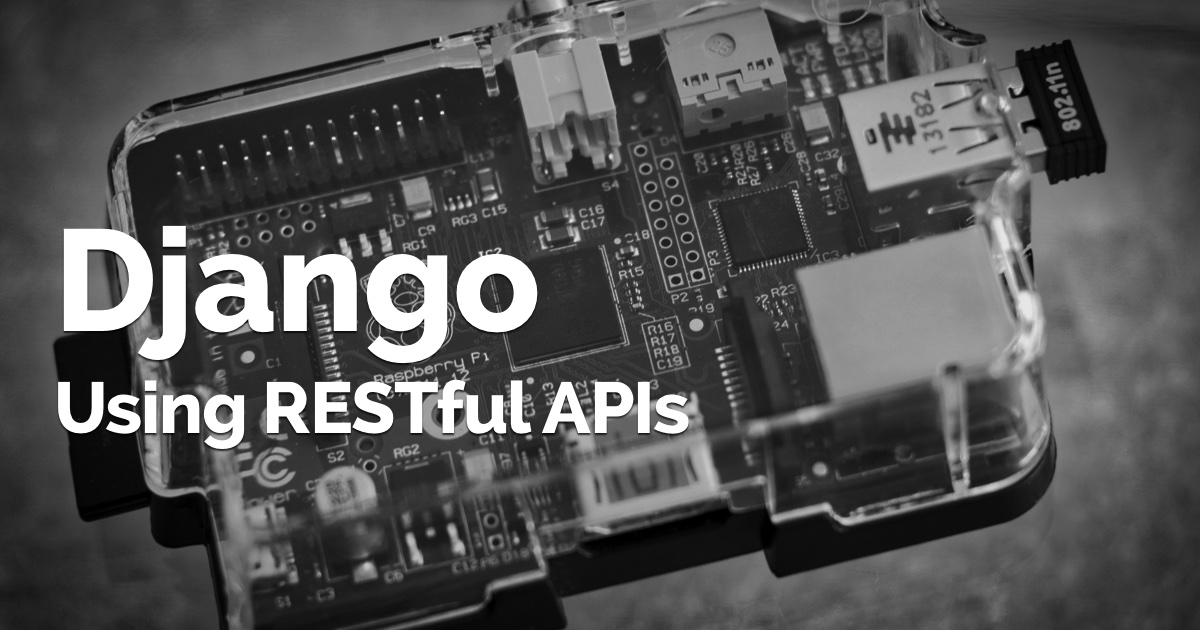
First, let’s get those terms out of our way. The REST acronym stands for Representational State Transfer, which is an architectural design. Usually when we use the term RESTful, we are referring to an application that implements the REST architectural design. API stands for Application Programming Interface, which is a software application that we interact programmatically, instead of using a graphical interface. In other words, we interact with it at a lower level at the source code, writing functions and routines.
tutorial
How to Implement Dependent/Chained Dropdown List with Django
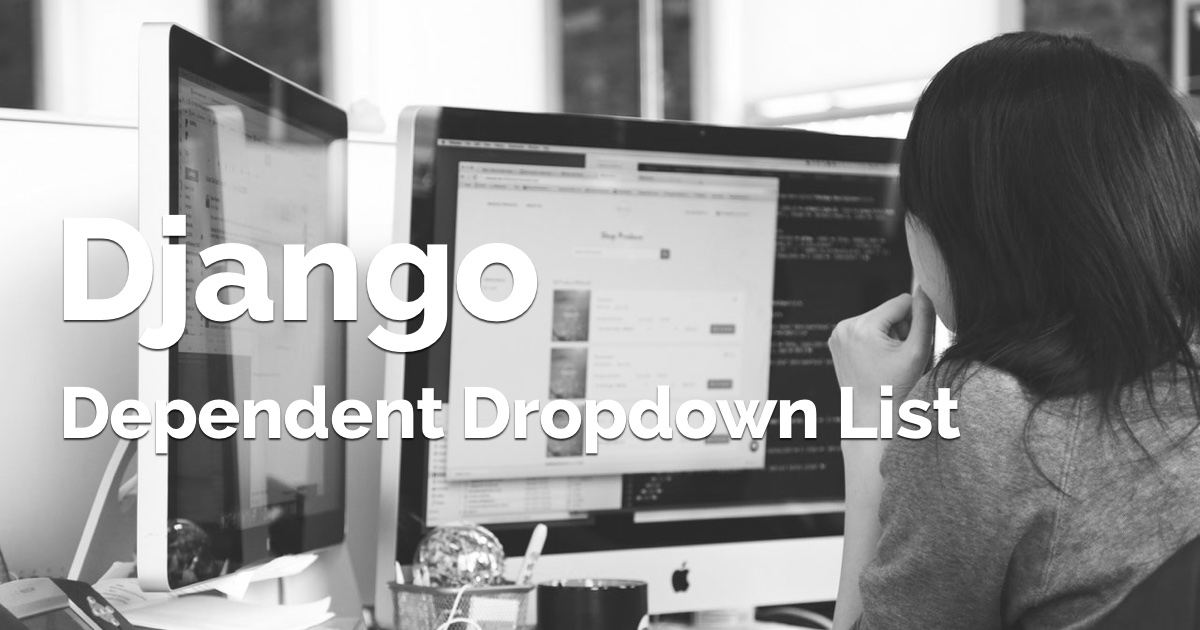
Dependent or chained dropdown list is a special field that relies on a previously selected field so to display a list of filtered options. A common use case is on the selection of state/province and cities, where you first pick the state, and then based on the state, the application displays a list of cities located in the state.
tutorial
How to Implement Multiple User Types with Django
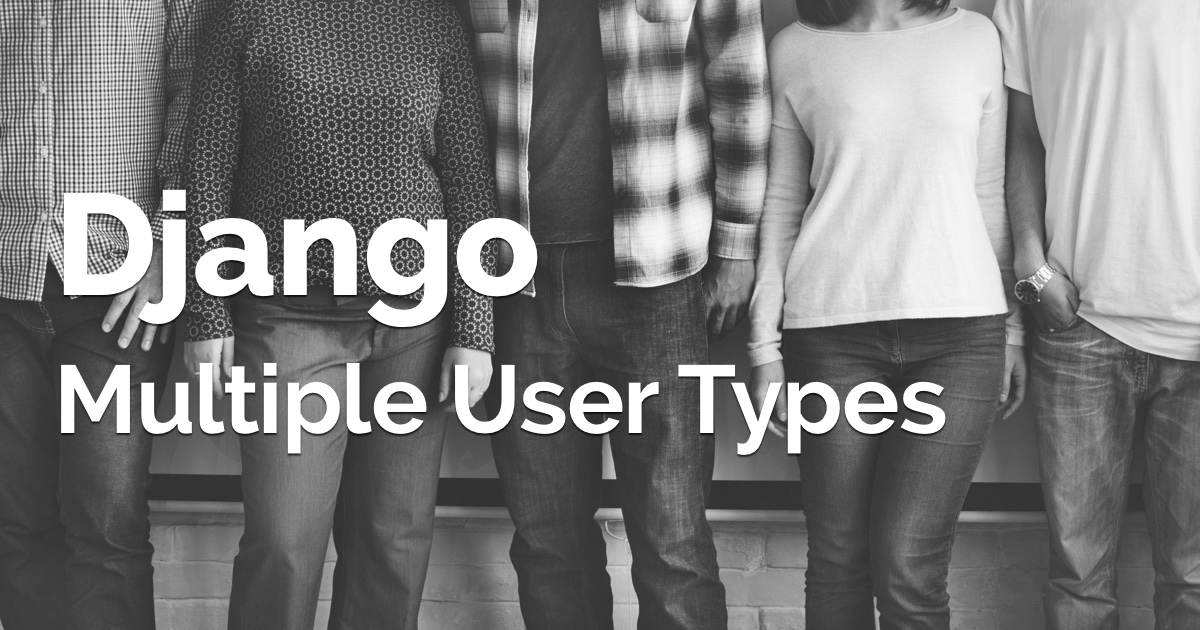
This is a very common problem many developers face in the early stages of the development of a new project, and it’s also a question I get asked a lot. So, I thought about sharing my experience with previous Django projects on how to handle multiple user types.
series
A Complete Beginner's Guide to Django - Part 7
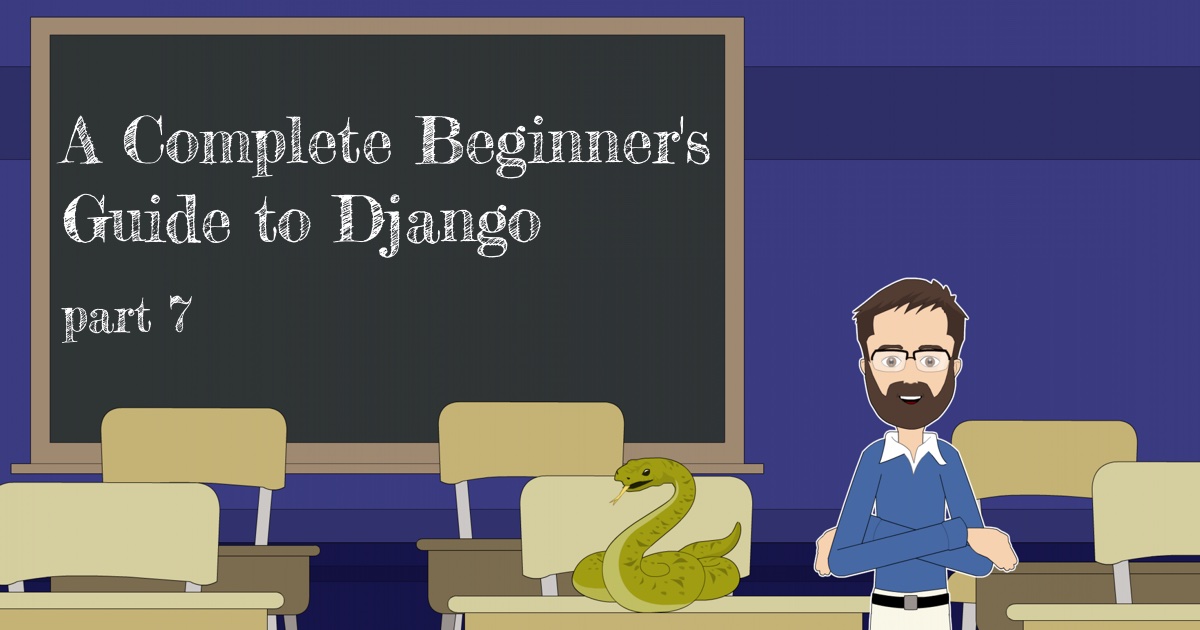
series
A Complete Beginner's Guide to Django - Part 6
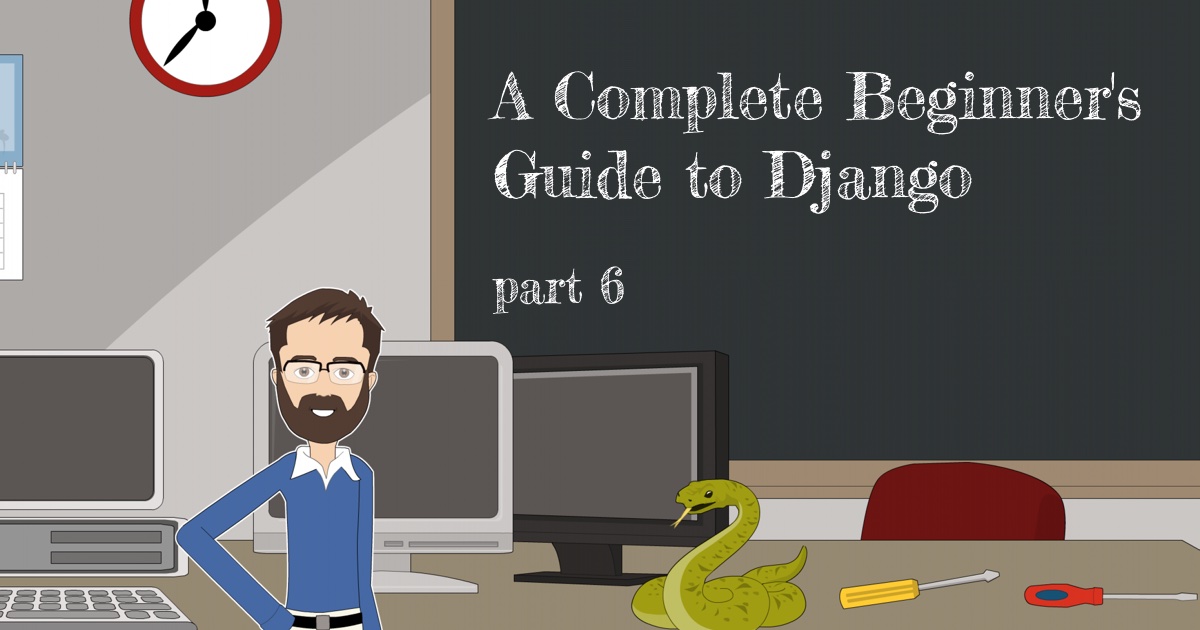
series
A Complete Beginner's Guide to Django - Part 5
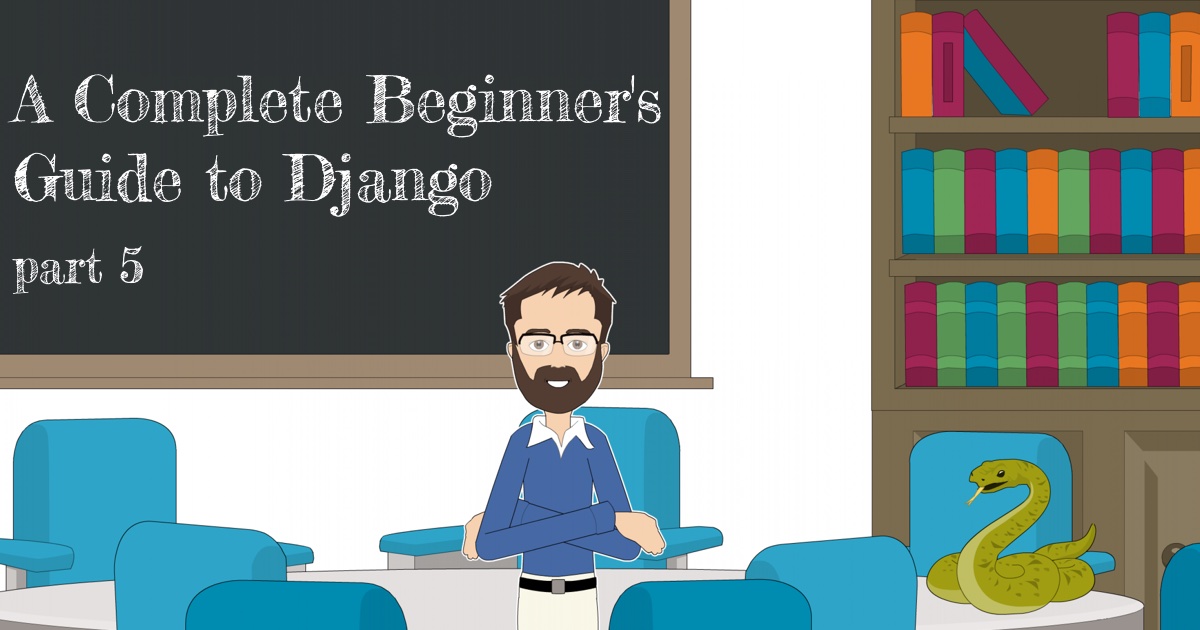
- ← Previous
- First page
- Page 2 of 11
- Last page
- Next →